Sending subscribe form on outer subscribe resource
Problem: Web-site has subscribe script that located on outer resource. After subscribe user hits the outside pages, and we need do that him to stay on current page.
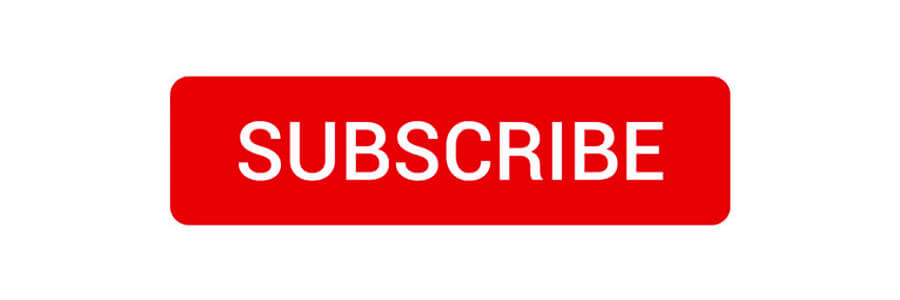
Solution: We'll use AJAX. Originally subscribe form looks so:
<form id="patientEducationForm" method="post" action="http://cl.exct.net/subscribe.aspx?lid=1234567" name="patientEducationForm">
<!-- BELOW 3 LINES CONTAINS THE PATH TO THE THANK YOU PAGE, ERROR PAGE AND THE ET MEMBER ID. ALL THREE ARE REQUIRED -->
<input type="hidden" name="thx" value="http://somesite.com/UCM_54321_RQI-Thank-You-page.jsp">
<input type="hidden" name="err" value="http://somesite.com/An-Error-occurred-with-your-submission_UCM_123345_Article.jsp">
<input type="hidden" name="MID" value="12345678">
Step 1: Edit the form (remove action, add nonce code):
<form id="patientEducationForm" method="post" name="patientEducationForm">
<!-- BELOW 3 LINES CONTAINS THE PATH TO THE THANK YOU PAGE, ERROR PAGE AND THE ET MEMBER ID. ALL THREE ARE REQUIRED -->
<input type="hidden" name="thx" value="http://somesite.com/UCM_54321_RQI-Thank-You-page.jsp">
<input type="hidden" name="err" value="http://somesite.com/An-Error-occurred-with-your-submission_UCM_123345_Article.jsp">
<input type="hidden" name="MID" value="12345678">
<script> var nonce = '<?php echo wp_create_nonce( 'send_form_nonce' ); ?>';</script>
...
<div id="response"></div>
...
Step 2: Add code to script:
function sendForm() {
var form = $( '#patientEducationForm' );
form.submit( function ( e ) {
var data = {
'action': 'sendform',
'query': form.serialize(),
'nonce_code': nonce,
}
$.ajax( {
url: ajax.url,
data: data,
type: form.attr( 'method' ),
success: function ( response ) {
$( '#response .success' ).remove();
$( '#response .error' ).remove();
$( '#response' ).append( response );
},
} );
e.preventDefault();
} );
}
Step 3: Send form from AJAX use cURL, write next code to functions.php
add_action( 'wp_ajax_sendform', 'send_form' );
add_action( 'wp_ajax_nopriv_sendform', 'send_form' );
function send_form() {
check_ajax_referer( 'send_form_nonce', 'nonce_code' );
$url = 'http://cl.exct.net/subscribe.aspx?lid=1234567';
$params = $_POST['query'];
$user_agent = "Mozilla/5.0 (compatible; MSIE 5.01; Windows NT 5.0)";
$ch = curl_init();
curl_setopt( $ch, CURLOPT_POST, 1 );
curl_setopt( $ch, CURLOPT_POSTFIELDS, $params );
curl_setopt( $ch, CURLOPT_URL, $url );
curl_setopt( $ch, CURLOPT_USERAGENT, $user_agent );
curl_setopt( $ch, CURLOPT_RETURNTRANSFER, 1 );
$result = curl_exec( $ch );
curl_close( $ch );
$message = '';
if ( strpos( $result, 'http://somesite.com/UCM_54321_RQI-Thank-You-page.jsp' ) ) {
$message = __( 'Please fill the fields above correctly', 'base' );
$message = '<p class="error">' . $message . '</p>';
} elseif ( strpos( $result, 'http://somesite.com/An-Error-occurred-with-your-submission_UCM_123345_Article.jsp' ) ) {
$message = __( 'Thank you for your submission', 'base' );
$message = '<p class="success">' . $message . '</p>';
}
echo $message;
wp_die();
}