Simple example use AJAX for HTML form
As they say: For developer doesn’t live by WordPress alone.Today, I give an example work to AJAX for building simple landing page without use most popular CMS. In this example we’ll not use back-end validation for fields, but we’ll use requirement attribute for fields. In really life, not by the example, I don’t recommended do it.
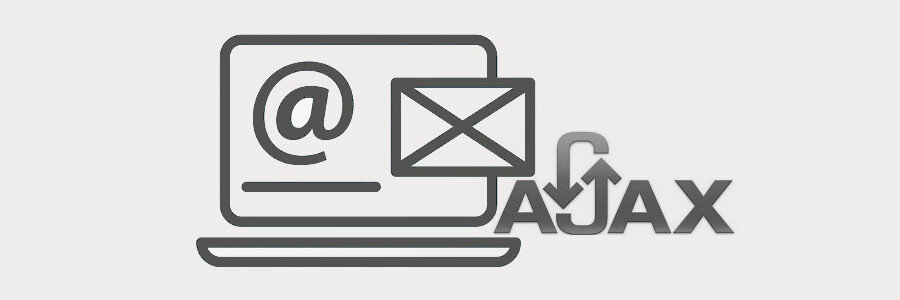
Problem: We need create simple HTML-form, that send emails to our mail box. Also, we'll not use WordPress. Only clean HTML.
Solution: We'll be use 3 files:
- index.html that contains content of the page, also we can use .php extension instead .html;
- mail.php that contain code send emails;
- script.js that contain JS-code.
NOTE: Yes, sure in addition we have jQuery library file and file of the styles, that I, as a matter of convenience put to relevant folders.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HelloAdmin Example</title>
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600;700;900&display=swap" rel="stylesheet">
<link media="all" rel="stylesheet" href="css/main.css">
<script src="http://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js" defer></script>
<script>window.jQuery || document.write( '<script src="js/jquery-3.3.1.min.js" defer><\/script>' )</script>
<script src="js/script.js" defer></script>
</head>
<body>
...
<form action="" class="contact-form" method="post">
<h1>Lorem ipsum dolor sit amet</h1>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
<div class="flex">
<div class="input">
<input type="text" name="name" placeholder="Name*" class="input-field" required>
</div>
<div class="input">
<input type="email" name="email" placeholder="Email*" class="input-field" required>
</div>
</div>
<div class="flex">
<div class="input">
<input type="text" name="campus" placeholder="Campus*" class="input-field" required>
</div>
<div class="input">
<input type="text" name="phone" placeholder="Phone" class="input-field">
</div>
</div>
<div class="flex">
<div class="input">
<textarea name="message" cols="10" rows="10" placeholder="Tell us a bit about yourself..." class="input-field"></textarea>
<button type="submit" name="submit" class="btn">Submit</button>
</div>
</div>
</form>
...
</body>
</html>
mail.php
<?php
$to = 'admin@yourdomain.com'; // Your e-mail address
$from = 'info@yourdomain.com'; // this is the sender's Email address
$name = $_POST['name'];
$email = $_POST['email'];
$campus = $_POST['campus'];
$phone = $_POST['phone'];
$form_message = $_POST['message'];
$message = "Hello! Somebody sent contact form on your site. Contact data: " . "\n\n" . "Name: " . $name . "\n\n" . "Email: " . $email . "\n\n" . "Campus: " . $campus . "\n\n" . "Phone: " . $phone . "\n\n" . "Message: " . $form_message;
$message2 = "Here is a copy of your message " . $name . "\n\n" . $form_message;
$subject = 'Message from HelloAdmin site';
$headers = "From:" . $from;
if ( mail( $to, $subject, $message, $headers ) ) {
mail( $email, $subject, $message2, $headers );
echo "<div class='success'>Mail Sent. Thank you " . $name . ", we will contact you shortly.</div>";
} else {
echo "<div class='error'>Error. Please try again.</div>";
}
?>
script.js
sendEmail();
function sendEmail() {
var form = jQuery( '.contact-form' );
jQuery( form ).on( 'submit', function ( e ) {
e.preventDefault();
jQuery.ajax( {
type: 'post',
url: 'mail.php',
data: form.serialize(),
success: function ( data ) {
jQuery( form ).trigger( 'reset' );
jQuery( data ).appendTo( form );
setTimeout( function () {
jQuery( '#contact-form .success, #contact-form .error' ).fadeOut( 300, function () {
jQuery( this ).remove();
} );
}, 5000 );
}
} );
} );
}
Sources:
Hurrah! After all I got a weblog from where I be able to truly take valuable data regarding my study and knowledge.