What about accessibility?
Web accessibility is great idea, especially, when your site targets a wide audience. In this article we’ll consider only small part from the series “increase accessibility sites” based on WordPress.
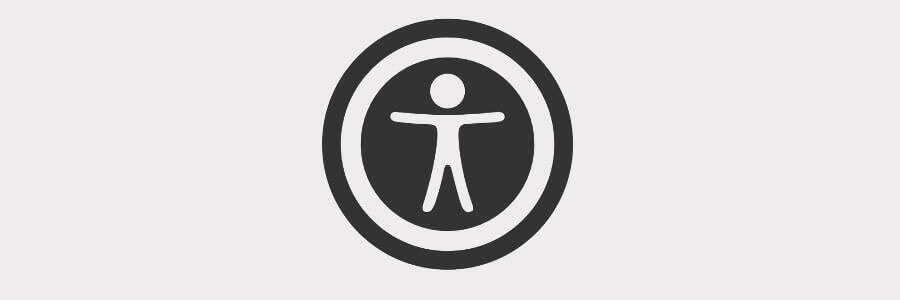
Problem: Increase web accessibility WordPress sites.
Solution: So, we'll tell about optimize images and tables. For images should be add new attribute role, e.g. role="presentation" and if you use function wp_get_attachment_image() that it can be done:
<?php
// Add Custom Attribute To Image In The Function wp_get_attachment_image() (for accessibility)
add_filter( 'wp_get_attachment_image_attributes', 'add_custom_attribute_to_image' );
function add_custom_attribute_to_image( $attr ) {
$attr['role'] = 'presentation';
return $attr;
}
As you can see it's simple. For tables our solution will less elegant:
<?php
// Add Custom Attribute To Table (for accessibility)
add_filter( 'the_content', 'custom_content_filter' );
function custom_content_filter( $content ) {
$current_post_type = get_post_type();
if ( ( $current_post_type === ( 'post' ) ) || ( basename( get_page_template() ) === 'page.php' ) ) {
$content = str_replace( '</th><th>', '</th><th scope="col">', $content );
$content = preg_replace( '/<tr><td>(.*?)<\/td>/', '<tr><th scope="row">$1</th>', $content );
}
return $content;
}
How you can see, in this example we added additional attributes only for post type post and for default page template. And small bonus:
<?php
add_filter( 'the_content', 'custom_content_filter' );
function custom_content_filter( $content ) {
$current_post_type = get_post_type();
if ( ( $current_post_type === ( 'post' ) ) || ( basename( get_page_template() ) === 'page.php' ) ) {
// For adaptive on mobiles used Bootstrap classes
$content = str_replace( '<table', '<div class="table-wrap"><table', $content );
$content = str_replace( '</table>', '</table></div>', $content );
$content = str_replace( '<table class="', '<table class="table', $content );
// Remove spaces in class names (w3c validator)
$content = preg_replace( '/class=["\']\s*(?P<class>[^"\'<>]+?)\s*["\']/i', 'class="$1"', $content );
// For accesability
$content = str_replace( '</th><th>', '</th><th scope="col">', $content );
$content = preg_replace( '/<tr><td>(.*?)<\/td>/', '<tr><th scope="row">$1</th>', $content );
}
return $content;
}